Here is the answer for your question
in Java Programming Language.
Kindly upvote if you find
the answer helpful.
NOTE : As you mentioned your
function sortByDistance will take in ArrayList as parameter, you
have to pass array list into the function. But I could see a
boolean passed to the function. If you expect the function to sort
according to the boolean value of increasing (i.e., if increasing
is true,sorts in increasing order, if false, sorts in decreasing
order)please find the below code.
Otherwise there is a buit-in
function sort() that automatically sorts an arraylist arranging its
elements in increasing order.
I will provide function
implementation using sort() and without using sort(). Please
comment below if you have any doubts.
####################################################################
CODE :
Using sort()
import java.util.ArrayList;
import java.util.Collections;
public class SortArrayList {
public static void main(String[] args){
//Have taken distances as double values
//Please change datatypes as per your requirements
ArrayList<Double> distances = new ArrayList<>();
//Adding distance values to arraylist
distances.add(90.5);
distances.add(88.6);
distances.add(190.6);
distances.add(23.6);
distances.add(48.6);
distances.add(289.6);
//Call method with increasing as true
SortArrayList obj = new SortArrayList();
obj.sortByDistance(distances, true);
System.out.println("After sorting in increasing order : ");
System.out.println(distances);
System.out.println("====================================");
obj.sortByDistance(distances, false);
System.out.println("After sorting in decreasing order : ");
System.out.println(distances);
System.out.println("====================================");
}
//As we have to take array list as parameter,had to change the
function prototype
public ArrayList sortByDistance(ArrayList<Double>
distances,boolean increasing) {
// can sort by distance increasing (true) or decreasing
(false)
if(increasing){
Collections.sort(distances);
}else{
double temp = distances.get(0);
//Sorting in decreading order (if increasing is false)
//NOTE : We cannot use sort() for decreasing order
for(int i = 0;i<distances.size();i++){
for(int j = i+1;j<distances.size();j++){
if(distances.get(i) < distances.get(j)){
temp = distances.get(i);
distances.set(i, distances.get(j));
distances.set(j,temp);
}
}
}
}
return distances;
}
}
|
###############################################################
SCREENSHOTS :
Please see the screenshots of the code below for the
indentations of the code.
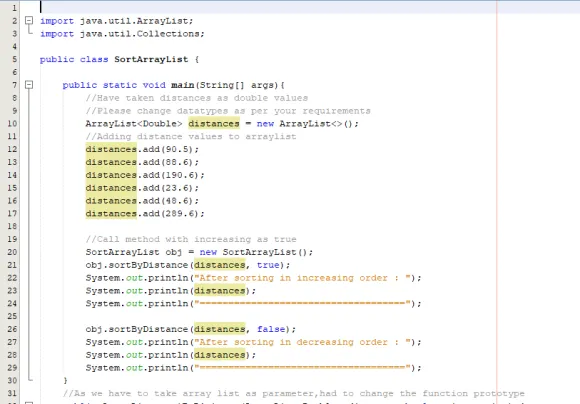
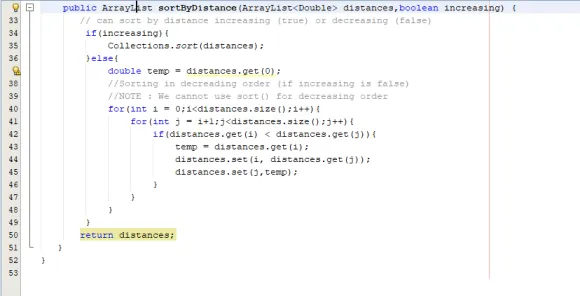
#################################################################
Without using sort()
import java.util.ArrayList;
import java.util.Collections;
public class SortArrayList {
public static void main(String[] args){
//Have taken distances as double values
//Please change datatypes as per your requirements
ArrayList<Double> distances = new ArrayList<>();
//Adding distance values to arraylist
distances.add(90.5);
distances.add(88.6);
distances.add(190.6);
distances.add(23.6);
distances.add(48.6);
distances.add(289.6);
//Call method with increasing as true
SortArrayList obj = new SortArrayList();
obj.sortByDistance(distances, true);
System.out.println("After sorting in increasing order : ");
System.out.println(distances);
System.out.println("====================================");
obj.sortByDistance(distances, false);
System.out.println("After sorting in decreasing order : ");
System.out.println(distances);
System.out.println("====================================");
}
//As we have to take array list as parameter,had to change the
function prototype
public ArrayList sortByDistance(ArrayList<Double>
distances,boolean increasing) {
// can sort by distance increasing (true) or decreasing
(false)
double temp = distances.get(0);
if(increasing){
for(int i = 0;i<distances.size();i++){
for(int j = i+1;j<distances.size();j++){
if(distances.get(i) > distances.get(j)){
temp = distances.get(i);
distances.set(i, distances.get(j));
distances.set(j,temp);
}
}
}
}else{
//Sorting in decreading order (if increasing is false)
//NOTE : We cannot use sort() for decreasing order
for(int i = 0;i<distances.size();i++){
for(int j = i+1;j<distances.size();j++){
if(distances.get(i) < distances.get(j)){
temp = distances.get(i);
distances.set(i, distances.get(j));
distances.set(j,temp);
}
}
}
}
return distances;
}
}
|
##########################################################################
SCREENSHOTS :
As the change is only with the function part while
comparing to above screenshots, sharing the screenshots of function
implementation.
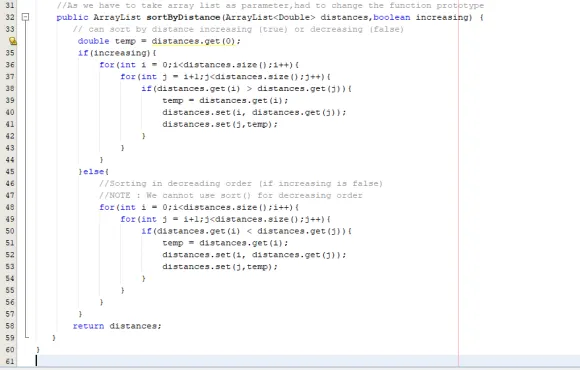
#####################################################################
OUTPUT :
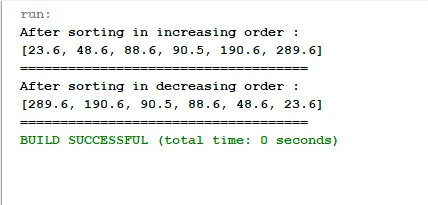
Any doubts regarding this can be explained with pleasure
:)