Answer-
Your code is given below in C
language-
- #include<stdio.h>
- int main()
- {
- int user_Num;
- int x;
- int i=1;
- printf("Enter the
inputs:\n");
- scanf("%d%d",&user_Num,&x); //take
input from user
- while(i<=3) //iterate the loop 3
times
- {
- user_Num = user_Num/x;
//perform division
- printf("%d ",user_Num);
//print the number
- i++; //increment the
iteration varilable by 1
- }
- return 0;
- }
Screenshot of running program and output-
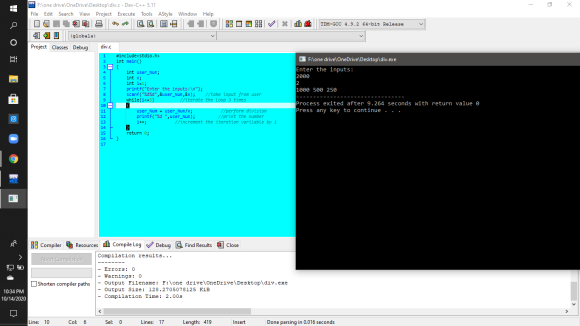
Note- Please do upvote, if any problem then comment in
box sure I will help.