HTML CODE:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link rel="stylesheet" href="./style.css">
<title>Math Quiz</title>
</head>
<body>
<section id="head-section">
<div
id="timer-container"><label>Timer: </label><div id="timer">30</div></div>
<h2>MATH QUIZ</h2>
<div
id="score-container"><label>Score: </label><div id="score">0</div></div>
</section>
<section id="question-section">
<div
id="question-container">
<div
id="question">36 x 15 = </div>
<input
type="number" name="answer" id="answer"><br>
</div>
<input type="button" name="submit" id="submit" value="Submit">
</section>
<script src="./script.js"></script>
</body>
</html>
CSS CODE (style.css):
body{
margin: 0;
font-family: "sans-serif", Arial;
}
#head-section{
height: 10vh;
display: flex;
justify-content: space-between;
background: #D63031;
justify-items: center;
align-items: center;
font-size: 20px;
color: white;
}
#timer-container,
#score-container{
margin: 0 10px;
display: flex;
}
#question-section{
height: 90vh;
background: #DAE0E2;
display: flex;
flex-direction: column;
text-align: center;
justify-content: space-around;
align-items: center;
}
#question-container{
display: flex;
justify-content: center;
}
#question{
padding: 0;
margin: 0;
font-size: 72px;
font-weight: bold;
}
#answer{
margin: 0 20px;
height: 80px;
font-size: 72px;
width: 300px;
text-align: center;
font-weight: bold;
}
#submit{
padding: 20px 30px;
font-size: 36px;
background: #019031;
color: white;
cursor: pointer;
border: none;
}
JAVASCRIPT CODE (script.js):
var score = 0;
var question =
1;
var timeLeft =
30; // In seconds
var op1 = 0;
var op2 = 0;
var intervalID =
0;
var timeoutID =
0;
document.getElementById("submit").addEventListener("click", checkAnswer);
function
nextQuestion(){
clearInterval(intervalID);
clearTimeout(timeoutID);
timeLeft = 30; // In
seconds
// If
number of questions is not more than 10
if(question <= 10){
// Get random operands for quiz
op1 =
Math.ceil(Math.random()*100);
op2 =
Math.ceil(Math.random()*100);
document.getElementById("question").innerHTML
= question.toString() + ") " +
op1.toString() + " x " +
op2.toString() + " = ";
document.getElementById("answer").value
= "";
// Start timer
intervalID =
setInterval(function(){
document.getElementById("timer").innerHTML
= timeLeft;
--timeLeft;
},
1000);
// Ask next question when user runs out of
time
timeoutID =
setTimeout(nextQuestion, 31*1000);
question++;
}
else{
alert("Quiz over! Your final score is " +
document.getElementById("score").innerText);
// Reset all values
question =
1;
score =
0;
document.getElementById("submit").disabled
= true;
document.getElementById("submit").style.background
= "lightgray";
}
}
// Check if answer provided by
the user is correct or not
function
checkAnswer(){
var response =
parseInt(document.getElementById("answer").value);
// If
answer is correct
if(op1*op2 == response){
score++;
document.getElementById("score").innerHTML
= score.toString();
}
// Ask
next question
nextQuestion();
}
// Ask next question when the
page loads completely
window.onload = nextQuestion;
SAMPLE OUTPUTS:
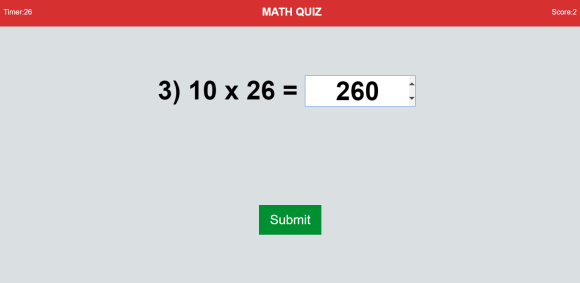
FOR ANY HELP JUST DROP A COMMENT