Here is the answer for your question
in Java Programming Language.
You can use a class (ex:
FoodStorage) to have all the details of a food set in one place and
you can create array of objects of the class as array of foodsets
and store data in the array.
I have used the above given
approach to store and search a food set by using the food set
name.
Kindly upvote if you find
the answer helpful. Please comment if you have any doubts. Thank
you.
CODE :
FoodStorage.java
public class FoodStorage {
//Required variables
private String setName;
private double cost;
private String mainCourse;
private String softDrink;
private String starters;
//Default constructor
FoodStorage(){ }
//Parameterized constructor
public FoodStorage(String setName, double cost, String mainCourse,
String softDrink, String starters) {
this.setName = setName;
this.cost = cost;
this.mainCourse = mainCourse;
this.softDrink = softDrink;
this.starters = starters;
}
//Getters
public String getSetName() { return setName; }
public double getCost() { return cost; }
public String getMainCourse() { return mainCourse; }
public String getSoftDrink() { return softDrink; }
public String getStarters() { return starters; }
//toString() to display contents of class object
@Override
public String toString() {
return setName + "\n$" + cost + "\n" + mainCourse + "\n" +
softDrink + "\n" + starters;
}
}
|
TestFoodStorage.java (This program is used to search a
food set)
import java.util.Scanner;
public class TestFoodStorage {
public static void main(String[] args){
//String to store setname given by user
String setName;
//Scanner object to read input from user
Scanner s = new Scanner(System.in);
//Create array for FoodStorage objects
FoodStorage[] foodsets = new FoodStorage[5];
//Store data in the array of FoodStorage objects
foodsets[0] = new FoodStorage("SET1",10,"Cheese
Burger","Coke","Fries");
foodsets[1] = new FoodStorage("SET2",14,"Spicy
Chicken","Juice","Potato");
foodsets[2] = new FoodStorage("SET3",10,"Veg Pizza","Lemon
Juice","Manchurian");
foodsets[3] = new FoodStorage("SET4",10,"Fried Rice","Pepsi","Aloo
fries");
foodsets[4] = new FoodStorage("SET5",10,"Veg Pulao","Orange
Juice","Cake");
//Display entire food menu
System.out.println("--------------------------");
System.out.println("Your entire food menu: ");
System.out.println("--------------------------");
//Loops thorugh every food set in foodsets array
for(FoodStorage foodset : foodsets){
//Prints the food set details
System.out.println(foodset);
System.out.println();
}
//Read the set name from the user in order to do a search
System.out.println("--------------------------");
System.out.print("Enter the set you want to search: ");
setName = s.nextLine();
//Call searchFoodSet method with setName given by user and foodsets
array as parameters
searchFoodSet(setName,foodsets);
}
//Implementation of searchFoodSet
public static void searchFoodSet(String setName,FoodStorage[]
foodsets){
//Create a flag and set it initially to false
boolean exists = false;
//Loop throug every food set in the foodsets array
for(FoodStorage foodset : foodsets){
//If set name of any food set matches given food set name
if(foodset.getSetName().compareToIgnoreCase(setName) == 0){
//Set the falg to true
exists = true;
//Print food set details
System.out.println("--------------------------");
System.out.println("Food Set Details");
System.out.println("--------------------------");
System.out.println(foodset);
}
}
//If the flag is false dsplay appropriate message
if(!exists){
System.out.println("--------------------------");
System.out.println("Food set " + setName + " has not been found in
the food storage.");
System.out.println("--------------------------");
}
}
}
|
SCREENSHOTS :
Please see the screenshots of the
code below for the indentations of the code.
FoodStorage.java
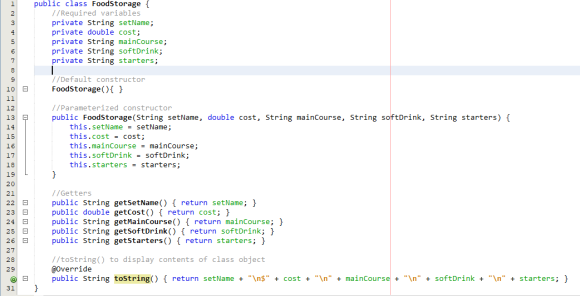
TestFoodStorage.java
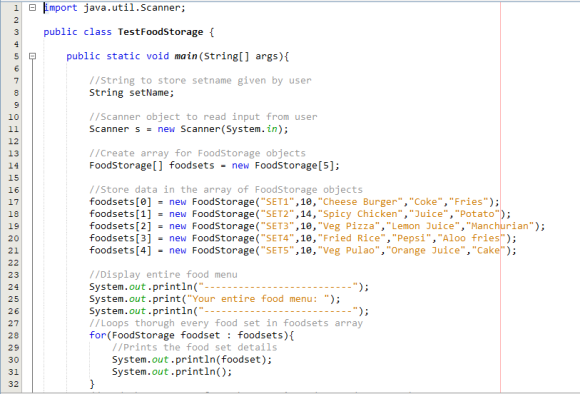
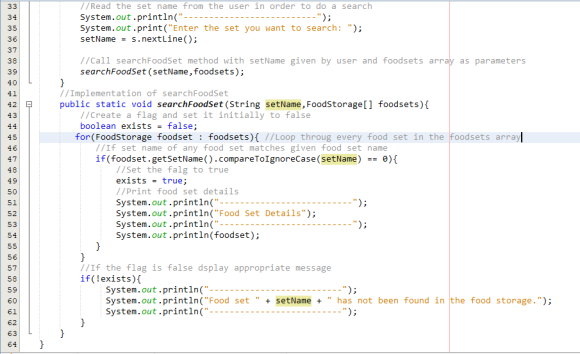
OUTPUT :
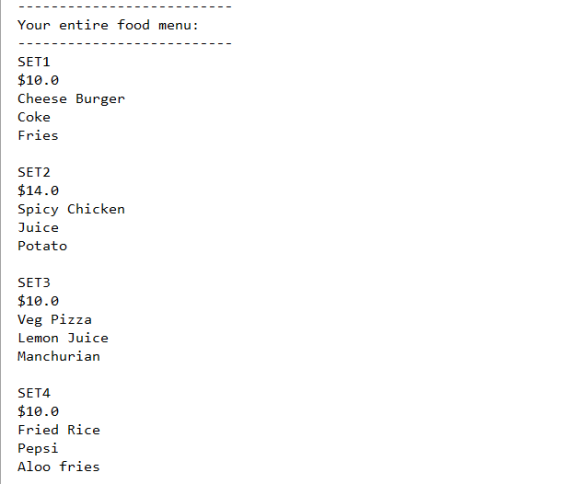
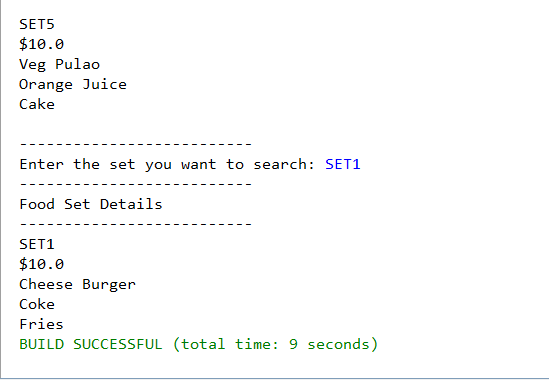
If a set name which is not
there in food storage is given,
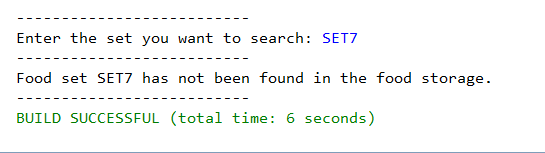
Any doubts regarding this
can be explained with pleasure :)