Here is the solution to
your question. I tried my best to solve your doubt, however, if you
find it is not as good as expected by you. Please do write your
further doubts regarding this question in the comment section, I
will try to resolve your doubts regarding the submitted solution as
soon as possible.
Please give proper indentation as shown in the screenshot
If you think, the solution provided by
me is helpful to you please do an upvote.
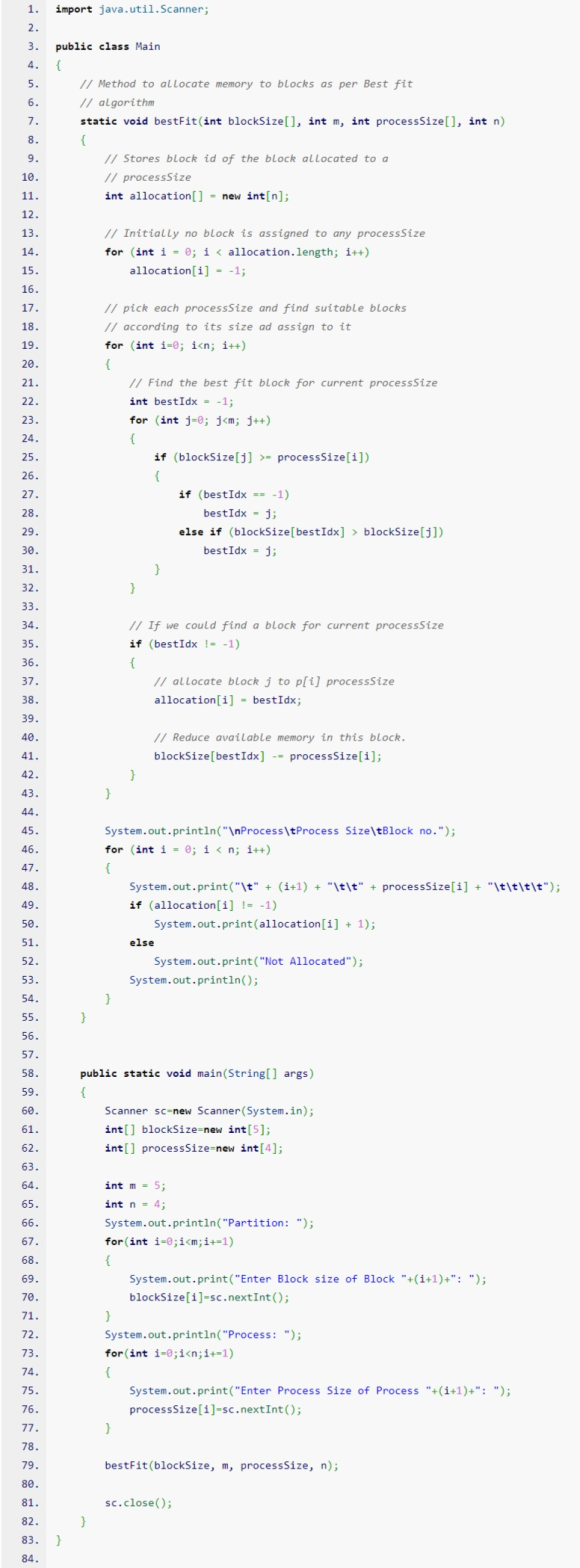 |
import java.util.Scanner;
public class Main
{
// Method to allocate memory to blocks as per Best
fit
// algorithm
static void bestFit(int blockSize[], int m, int
processSize[], int n)
{
// Stores block id of the block
allocated to a
// processSize
int allocation[] = new
int[n];
// Initially no block is assigned
to any processSize
for (int i = 0; i <
allocation.length; i++)
allocation[i] =
-1;
// pick each processSize and find suitable
blocks
// according to its size ad assign
to it
for (int i=0; i<n; i++)
{
// Find the best
fit block for current processSize
int bestIdx =
-1;
for (int j=0;
j<m; j++)
{
if (blockSize[j] >= processSize[i])
{
if (bestIdx == -1)
bestIdx =
j;
else if (blockSize[bestIdx]
> blockSize[j])
bestIdx =
j;
}
}
// If we could
find a block for current processSize
if (bestIdx !=
-1)
{
// allocate block j to p[i] processSize
allocation[i] = bestIdx;
// Reduce available memory in this block.
blockSize[bestIdx] -= processSize[i];
}
}
System.out.println("\nProcess\tProcess Size\tBlock no.");
for (int i = 0; i < n;
i++)
{
System.out.print("\t" + (i+1) + "\t\t" + processSize[i] +
"\t\t\t\t");
if
(allocation[i] != -1)
System.out.print(allocation[i] + 1);
else
System.out.print("Not Allocated");
System.out.println();
}
}
public static void main(String[] args)
{
Scanner sc=new Scanner(System.in);
int[] blockSize=new int[5];
int[] processSize=new int[4];
int m = 5;
int n = 4;
System.out.println("Partition: ");
for(int i=0;i<m;i+=1)
{
System.out.print("Enter Block size of Block "+(i+1)+": ");
blockSize[i]=sc.nextInt();
}
System.out.println("Process: ");
for(int i=0;i<n;i+=1)
{
System.out.print("Enter Process Size of Process "+(i+1)+":
");
processSize[i]=sc.nextInt();
}
bestFit(blockSize, m, processSize,
n);
sc.close();
}
}
|
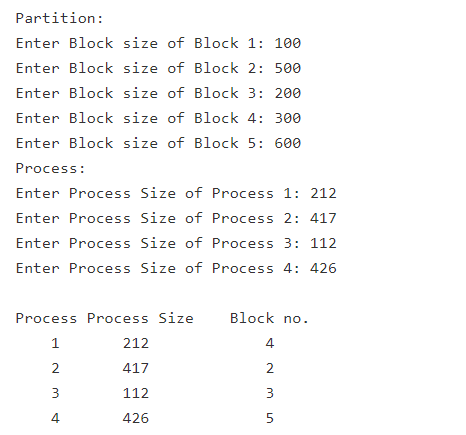 |