Answer-
Your code and output is given below as your requirement
-
- #include <iostream>
- using namespace std;
- int main()
- {
- cout<<"\t**********Information of Hotal that show some
statistics **********\n";
-
- const int numberof_FLOORS = 1;
- const int numberof_ROOMS = 10;
- int floors;
- int rooms; //variable define
- int occupied;
- int totalRooms=0, totalOccupied=0;
-
- cout<<" Enter the number of floors,\n";
- do{
- cout<<"(Do not accept a number less than
"<<numberof_FLOORS
- <<")\n";
- cout<<"Number of floors: ";
- cin >>floors;
- }while(floors<numberof_FLOORS); // input validation
-
-
cout<<"****************************************************\n";
-
-
- for(int floor=1; floor <= floors; floor++){
-
- if(floor == 13){ //// Loop over all the floors to get the
data.
- continue;
- }
-
-
- cout<<"Enter the number of rooms in floor \""
- <<floor<<",\"\n"; //// Get the number of rooms in
each floor,
- do{
- cout<<"(Do not accept a number less than
"<<numberof_ROOMS
- <<")\n";
- cout<<"Number of rooms: ";
- cin >>rooms;
- }while(rooms<numberof_ROOMS);
-
- totalRooms += rooms;
-
-
cout<<"*************************************************\n";
-
- cout<<"Enter the number of occupied rooms,\n";
- do{
- cout<<"(Do not accept a number less than 0)\n";
- cout<<"(it also could not be more than
"<<rooms<<"\n";
- cout<<"Number of occupied rooms: ";
- cin >>occupied;
- }while(occupied<0 || occupied>rooms);
-
- totalOccupied += occupied;
-
-
cout<<"**************************************************\n";
- }
-
- //
---------------------------------------------------------
- // Display Information of Hotal that show some statistics
- cout<<"Display Information of Hotal that show some
statistics\n";
- cout<<" Total number of rooms:
"<<totalRooms<<std::endl;
- cout<<" Total number of occupied:
"<<totalOccupied
- <<std::endl;
- cout<<"Total number of free ones: "
- <<(totalRooms-totalOccupied)<<std::endl;
- cout<<" Percentage of occupied: "
- <<1.0*totalOccupied/totalRooms<<std::endl;
-
-
cout<<"********************************************************\n";
- return 0;
- }
-
Screenshot of output-
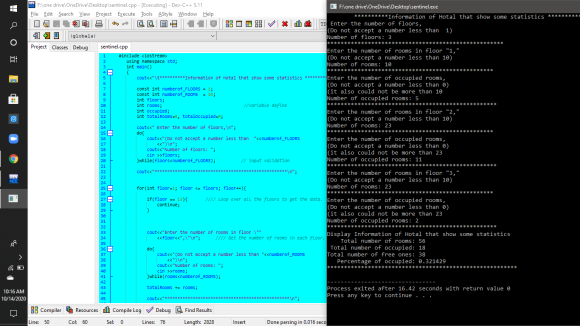
Note- Please do upvote, if any problem then comment in
box sure I will help.